Navigate between pages
# SUI Swap SUI Swap is an Automated Market Maker (AMM) protocol built on the SUI blockchain, utilizing the constant product model (x*y=k) for token swaps. This project implements a complete set of Decentralized Exchange (DEX) core functionalities, enabling users to add liquidity and perform token swaps. ## Core Features - **Liquidity Pool Management**: Create and manage trading pairs between any two tokens - **Add/Remove Liquidity**: Users can add liquidity to receive LP tokens and burn LP tokens to retrieve original tokens - **Token Swaps**: Efficient token exchange based on constant product formula - **Fee Mechanism**: Configurable transaction fees for each trade, rewarding liquidity providers - **LP Token Value Calculation**: Support for calculating real-time value and profit of LP tokens ## Project Architecture The project consists of four main modules: - `factory.move`: Factory contract responsible for creating and registering liquidity pools - `liquidity_pool.move`: Liquidity pool implementation, managing token reserves and swap logic - `swap.move`: Swap logic and price calculation - `sui_swap.move`: User interface functions ## Permission Design This project implements secure permission control mechanisms: - **Liquidity Pool Creation**: Only factory owner can create new liquidity pools, ensuring quality control - **Fee Management**: Only factory owner can update default transaction fees - **Ownership Transfer**: Factory owner can transfer ownership to a new address ## User Guide 1. **Create Factory**: - Factory contract is automatically created during initialization - Initial deployer becomes the factory owner - Default fee rate is 0.3% 2. **Create Liquidity Pool**: - Only factory owner can create new liquidity pools - Supports two creation methods: - Create with custom fee rate - Create with default fee rate (0.3%) - Initial token amounts are required for creation 3. **Add Liquidity**: - Any user can add liquidity to existing pools - Receive LP tokens proportional to contribution - Automatic handling of mismatched token amounts with remaining tokens returned - Supports adding tokens in any ratio 4. **Token Swaps**: - Supports bidirectional token swaps (X->Y and Y->X) - Users can perform token swaps in liquidity pools - Supports setting minimum output amount and deadline - Automatic slippage protection - Automatic rollback on transaction failure 5. **Remove Liquidity**: - LP token holders can remove liquidity at any time - Receive original tokens and accumulated fees proportionally - Supports partial or complete removal 6. **LP Token Value Calculation**: - Calculate token value for any amount of LP tokens - Calculate profit for LP token holders 7. **Factory Management**: - Factory owner can update default transaction fees - Supports factory ownership transfer - View all created liquidity pools ## Technical Features - **Large Number Handling**: Uses u128 for large number calculations to prevent integer overflow - **Precise Calculations**: Implements precise mathematical calculations to avoid rounding errors - **Event System**: Complete event logging for off-chain tracking and analysis - **Type Safety**: Strict type checking ensures transaction safety ## Security Considerations - Precise mathematical calculations to prevent overflow and rounding errors - Permission checks for all critical operations - Comprehensive test coverage ensures functional correctness - Uses u128 for large number calculations to prevent integer overflow - Strict type checking and boundary condition validation - Complete error handling mechanism
The women_saloon module on sui blockchain platform offers a comprehensive system for managing women saloon. it allow users to 1. Create saloon. 2. Add services to the saloon. 3. Pay for services. 4. Rate, comment on the saloon. 5. Withdraw funds and more.
The Skill Exchange Platform is a decentralized application built on the Sui blockchain that facilitates the exchange of skills between users. It allows users to register, offer their skills, book services, and provide reviews. This README provides a comprehensive guide for new users to understand the project, set it up on their local machine, and run the application.
TimeLockDAO is a blockchain-based transaction scheduling framework designed to enable delayed execution of transactions in a secure and controlled manner. This module ensures that transactions are executed only when specific conditions are met, offering flexibility and reliability for time-sensitive operations on decentralized platforms. Key Use Cases: Automated Transactions: Schedule recurring or one-time transactions to execute at specific times. Time-Locked Operations: Enforce delays for sensitive operations, enhancing security. Conditional Executions: Combine with smart contract logic to enable transactions that depend on external conditions. TEST: TestTimelockDAO is a Move module designed to test the core functionalities of the TxScheduler module. The TxScheduler module implements a basic timelock mechanism for scheduling, executing, and canceling transactions. This test module ensures the correctness of these functionalities through various test scenarios. Features The module provides the following test functionalities: Initialization: Verify that the scheduler initializes correctly with an empty transaction map. Transaction Queueing: Test the ability to queue transactions and ensure they are stored properly in the timelock state. Timestamp Validation: Ensure that transactions with invalid timestamps (e.g., timestamps in the past) are not queued. Transaction Execution: Test the ability to execute queued transactions after their timelock has expired. Transaction Cancellation: Validate that transactions can be canceled by their owners and removed from the timelock state. Unauthorized Cancellation: Ensure that transactions cannot be canceled by non-owners. Module Overview Functions 1. create_tx_data Creates a dummy TxData structure for testing. Parameters: owner: Address of the transaction owner. target: Target address of the transaction. function_name: Function name to be executed. data: Data payload for the transaction. timestamp: Scheduled timestamp for execution. 2. test_initialize Tests the initialization of the TxScheduler module to ensure it starts with an empty transaction map. 3. test_queue_tx Tests queuing a transaction and verifies that the transaction is successfully added to the timelock state. 4. test_queue_invalid_timestamp Tests that transactions with timestamps in the past are not allowed to be queued. 5. test_execute_tx Tests the execution of a valid transaction after the timelock duration has passed. Verifies that the transaction is removed from the state. 6. test_cancel_tx Tests the cancellation of a transaction by its owner. Ensures that the transaction is removed from the state. 7. test_cancel_tx_non_owner Tests that a transaction cannot be canceled by a non-owner, ensuring proper ownership enforcement. Usage To run the tests provided in this module, use the Test framework in the Move environment. Follow these steps: Compile the Module: Ensure the TxScheduler module is available in your project, and compile the TestTimelockDAO module: move build Run Tests: Execute the test suite: move test Queuing a Transaction This test ensures that a transaction can be successfully queued: let mut state = TxScheduler::initialize(@0x1); let tx_id = b"tx1"; let current_time = 100; let tx_data = create_tx_data(@0x1, @0x2, b"test_func", b"test_data", current_time + 20); let queued = TxScheduler::queue_tx(&mut state, tx_id, tx_data, current_time); Test::assert(queued, 1); Test::assert(table::contains(&state.tx_map, tx_id), 2); Canceling a Transaction by Non-Owner This test ensures only the owner of a transaction can cancel it: let mut state = TxScheduler::initialize(@0x1); let tx_id = b"tx5"; let current_time = 100; let tx_data = create_tx_data(@0x1, @0x2, b"test_func", b"test_data", current_time + 20); let _ = TxScheduler::queue_tx(&mut state, tx_id, tx_data, current_time); let canceled = TxScheduler::cancel_tx(&mut state, tx_id, @0x3); Test::assert(!canceled, 8);
A simple Sui Move stream payment-based payroll system. ## Introduction + This module allows anyone to send micro-payments per time interval to another user. + After a `Payment` is created, the `Payee` can start the payment by providing the recipient's address. + `Payment` is then stored in a shared object `Payments` and capabilities (`PayeeCap` and `PayerCap`) for access control. + The `PayerCap` has privilages like `pause_payment`, `resume_payment` and `cancel_payment`. + The `PayeeCap` has the privilege of `withdraw_payment`. This takes into account the amount that has already been withdrawn. + Composability and convenience were put into consideration when creating functions and structs. ## Core Sturcts 1. Payments + `Payments` is a shared object that keeps information like fee rates, supported tokens, and the balance of fees collected. + Also when a payment is active, Its `Payment` struct is dynamically linked to this shared object. 2. Payment + `Payment` is an object created by a payer. It stores the balance to be "streamed" along with time-related variables to take track of how much has been "streamed". 3. PayerCap + `PayerCap` is an access control for the payer. 4. PayeeCap + `PayeeCap` is an access control for the recipient. 5. AdminCap + `AdminCap` has the authority to alter the `Payments` object. + It can change fee rates, withdraw fee balance, and add a new coin to the whitelist. ## Core function 1. create_payment<COIN-TYPE> + Create a `Payment` struct. + Input with `Coin`, `duration` (how long you want to stream), `payments` + Note: `Coin`: balance you want to stream + fee (default 0.3%). 2. start_payment<COIN-TYPE> + starts a `Payment` and sends `PayeeCap` to `Recipient address`. + Input with `Payment` `Recipient address` `&Clock` `&mut Payments` + Output: `PayerCap`. 3. pause_payment<COIN-TYPE> + pause a `Payment`. + permission: user with `PayerCap` 4. resume_payment<COIN-TYPE> + Resume a pause `Payment`, fails in `Payment` status in not `PAUSED`. + permission: user with `PayerCap` 5. cancel_payment<COIN-TYPE> + cancel a `Payment`, fails in `Payment` status in already `CANCELLED`. + Returns: "unstreamed" `Coin<COIN-TYPE>` + permission: user with `PayerCap` 4. withdraw_payment<COIN-TYPE> + withdraw stream coins from `Payment`. + can be run multiple times. + Keep account of how much has been withdrawn. + Returns: "streamed" `Coin<COIN-TYPE> + permission: user with `PayeeCap`. 5. set_fee_percent<COIN-TYPE> + Change current fee rates. + permission: user with `AdminCap` 6. Admin_withdraw<COIN-TYPE> + withdraw a from fee balance. + permission: user with `AdminCap`
Farm365 is a farm equipment store and rentals services build on sui blockchain. The platform incorporate several features such as creating farm, adding equipment to the farm for rent and the return of rented item, purchase, withdraw funds and more. This module leverages blockchain technology to ensure transparency, security, and efficiency in farm rental businesses.
This module implements the SwapDex protocol, which provides a decentralized exchange (DEX) with support for liquidity pools, limit orders, market orders, loyalty rewards, and staking functionalities. It is built on the Sui blockchain and integrates with DeepBook for pool and order management. ## Key Features 1. **Token Minting:** Mint WBTC, WETH, and SWAPDEX tokens. 2. **Pool Creation:** Create and manage liquidity pools for token trading. 3. **Order Management:** Place limit and market orders for base and quote assets. 4. **Rewards System:** Earn loyalty points and DEX token rewards for trades. 5. **Staking:** Stake SWAPDEX tokens to earn points and enhance loyalty rewards. 6. **NFT Rewards:** Redeem loyalty points for exclusive NFTs. ### **Reward and Loyalty Mechanism** - **Earning Points**: Users earn 1 point for every trade if their loyalty account has a non-zero stake. - **DEX Token Rewards**: Users are rewarded with 1 SWAPDEX token for trades. - **Redeeming Points**: Users can redeem 10 points to mint an NFT as a reward. --- ## Constants - **`FLOAT_SCALING`**: Scaling factor for fractional values (`1_000_000_000`). - **`ENeeds10Points`**: Error code indicating insufficient points for NFT rewards (`0`). --- ## Core Data Structures ### `SWAPDEX` - Represents the SWAPDEX token. - Has the `drop` capability. ### `SwapEvent` - Emitted during successful swaps. - **Fields**: - `message`: A vector of bytes containing event details. ### `RewardEvent` - Emitted when a user is rewarded with DEX tokens. - **Fields**: - `message`: A vector of bytes containing event details. ### `LoyaltyAccount` - Tracks user loyalty points and staked tokens. - **Fields**: - `id`: Unique identifier. - `stake`: Staked balance in SWAPDEX tokens. - `points`: Accumulated loyalty points. ### `NFT` - Represents a non-fungible token rewarded to loyal users. - **Fields**: - `id`: Unique identifier. --- ## Functions ### Initialization #### `init(witness: SWAPDEX, ctx: &mut TxContext)` Initializes the SWAPDEX token by creating a treasury cap and metadata. --- ### Token Minting #### `mint_weth(cap: &mut TreasuryCap<WETH>, amount: u64, ctx: &mut TxContext)` Mints WETH tokens and transfers them to the caller. #### `mint_wbtc(cap: &mut TreasuryCap<WBTC>, amount: u64, ctx: &mut TxContext)` Mints WBTC tokens and transfers them to the caller. --- ### Pool Management #### `new_pool<WBTC, WETH>(payment: &mut Coin<SUI>, ctx: &mut TxContext)` Creates a new liquidity pool for WBTC and WETH. #### `new_custodian_account(ctx: &mut TxContext)` Creates a new custodian account for managing assets. #### `make_base_deposit<WBTC, WETH>(pool, coin, account_cap)` Deposits a base asset into a liquidity pool. #### `make_quote_deposit<WBTC, WETH>(pool, coin, account_cap)` Deposits a quote asset into a liquidity pool. #### `withdraw_base<BaseAsset, QuoteAsset>(pool, quantity, account_cap, ctx)` Withdraws base assets from a pool. #### `withdraw_quote<WBTC, WETH>(pool, quantity, account_cap, ctx)` Withdraws quote assets from a pool. --- ### Order Management #### `place_ask_order<WBTC, WETH>(...)` Places a limit order to sell a base asset for a quote asset. #### `place_bid_order<WBTC, WETH>(...)` Places a limit order to buy a base asset with a quote asset. #### `place_base_market_order<WBTC, WETH>(...)` Executes a market order to trade a base asset. #### `place_quote_market_order<WBTC, WETH>(...)` Executes a market order to trade a quote asset. #### `swap_exact_base_for_quote<WBTC, WETH>(...)` Swaps a specific amount of base asset for a quote asset. #### `swap_exact_quote_for_base<WBTC, WETH>(...)` Swaps a specific amount of quote asset for a base asset. --- ### Loyalty System #### `create_account(ctx: &mut TxContext) -> LoyaltyAccount` Creates a new loyalty account. #### `loyalty_account_stake(account: &LoyaltyAccount) -> u64` Returns the staked balance in a loyalty account. #### `loyalty_account_points(account: &LoyaltyAccount) -> u64` Returns the loyalty points of an account. #### `get_reward(account: &mut LoyaltyAccount, ctx: &mut TxContext) -> NFT` Redeems 10 loyalty points for an NFT. #### `stake(account: &mut LoyaltyAccount, stake: Coin<SWAPDEX>)` Stakes SWAPDEX tokens into a loyalty account. #### `unstake(account: &mut LoyaltyAccount, ctx: &mut TxContext) -> Coin<SWAPDEX>` Unstakes SWAPDEX tokens from a loyalty account. --- ### Reward System #### `reward_user_with_dex(cap: &mut TreasuryCap<SWAPDEX>, ctx: &mut TxContext)` Grants a reward of 1 SWAPDEX token to the user. --- ### Event Emission #### `SwapEvent` Emitted after a successful swap operation. #### `RewardEvent` Emitted when a user receives DEX token rewards. This module is a robust example of integrating a loyalty program with a DEX while leveraging the Sui blockchain's capabilities for high-performance token swaps and decentralized finance operations. It balances functionality, usability, and rewards to encourage user participation.
Sui-Move-GigSphere is a smart contract designed to power a decentralized gig platform on the Sui blockchain. It simplifies managing gigs, users, and payments by enabling the creation of a gig manager, user registration, and features like posting, assigning, and completing gigs. It also supports funding user accounts and tracking key events, ensuring transparency and efficiency throughout the gig lifecycle.
# `joy_swap` Module The `joy_swap` module provides a decentralized finance (DeFi) application where users can create liquidity pools, add liquidity, remove liquidity, and swap tokens. This module supports operations involving two different types of tokens, `Coin_A` and `Coin_B`, in a liquidity pool. ## Structs ### 1. **LP<phantom Coin_A, phantom Coin_B>** + This struct represents a liquidity pool's LP (Liquidity Provider) token. + It is a phantom struct that pairs two types of coins (`Coin_A` and `Coin_B`) together to create an LP token for the pool. ### 2. **Pool<phantom Coin_A, phantom Coin_B>** + This struct represents a liquidity pool containing two types of tokens (`Coin_A` and `Coin_B`). + It holds the balances of each token and tracks the LP token supply for the pool. --- ## Functions ### 1. **Create Pool** - **Description**: Users can create a new liquidity pool containing two different tokens: `Coin_A` and `Coin_B`. When creating the pool, the user must provide an amount of `Coin_A` and `Coin_B`. - **How it Works**: 1. The user calls the `create_pool<Coin_A, Coin_B>` function with the two tokens `Coin_A` and `Coin_B`. 2. The function verifies that both tokens have non-zero values. 3. The values of the tokens are converted into `Balance` types, and the number of liquidity pool (LP) tokens to mint is calculated based on the square root of the product of the two token values. 4. The pool is created and the LP tokens are issued to the user. - **Related Event**: - `PoolCreated`: Emits an event containing the pool's ID, token types, initial token values, and minted LP tokens. ### 2. **Add Liquidity** - **Description**: Users can add liquidity to an existing liquidity pool. The user provides additional amounts of `Coin_A` and `Coin_B`. If the amounts do not match the existing pool ratios, excess tokens are refunded to the user. - **How it Works**: 1. The user calls the `add_liquidity<Coin_A, Coin_B>` function, passing the liquidity pool and the tokens to add. 2. The function verifies that both tokens have non-zero values and calculates the current ratios of the pool's tokens. 3. If the provided tokens do not match the pool's ratio, the excess tokens are refunded. 4. The LP token supply is adjusted based on the new liquidity added. 5. The newly minted LP tokens are issued to the user. - **Related Event**: - `LiquidityAdded`: Emits an event containing the pool's ID, token types, added token values, and minted LP tokens. ### 3. **Remove Liquidity** - **Description**: Users can remove liquidity from an existing liquidity pool by providing LP tokens. The system calculates the amounts of `Coin_A` and `Coin_B` to be refunded based on the LP token value and the pool's ratio. - **How it Works**: 1. The user calls the `remove_liquidity<Coin_A, Coin_B>` function with the LP token to burn. 2. The function verifies that the LP token has a non-zero value. 3. The liquidity to be removed is calculated based on the LP token value and the pool's token ratios. 4. The LP tokens are burned, and the corresponding amounts of `Coin_A` and `Coin_B` are returned to the user. - **Related Event**: - `LiquidityRemoved`: Emits an event containing the pool's ID, token types, removed token values, and burned LP tokens. ### 4. **Swap Tokens A to B** - **Description**: This function allows users to swap `Coin_A` for `Coin_B`. The swap follows the constant product formula: `k = balance_a * balance_b`. Fees are deducted from the output token. - **How it Works**: 1. The user calls the `swap_a_to_b<COin_A, Coin_B>` function with `Coin_A` to swap. 2. The function calculates the new balance after the swap and ensures that the new token value is within the expected range. 3. A small fee (0.3%) is deducted from the output token (`Coin_B`). 4. The swapped tokens are transferred to the user. - **Related Event**: - `Swaped`: Emits an event containing the pool's ID, the input token, input value, output token, and output value. ### 5. **Swap Tokens B to A(same as above)** --- ## Test 
I created a toolkit with token release, object tokenized, and object SFT compositing
The Sui-Voucher is a blockchain-based smart contract for managing vouchers, users, and funds using the Sui Move language. It allows decentralized operations such as creating a voucher manager, registering users with unique IDs, issuing vouchers, funding the manager, and redeeming vouchers for SUI coins. The system logs key actions through events, ensuring transparency, fraud prevention, and accountability.
module DeFiChallenge::LendingPlatform { use sui::transfer; use sui::object; use sui::tx_context::{Self, TxContext}; use sui::coin::{Self, Coin}; use sui::balance::Balance; struct Loan { id: u64, borrower: address, lender: address, amount: u64, collateral: Coin<Balance>, interest_rate: u64, duration: u64, start_time: u64, } public fun request_loan( borrower: address, collateral: Coin<Balance>, loan_amount: u64, interest_rate: u64, duration: u64, ctx: &mut TxContext ): Loan { // Generate a unique ID for the loan let loan_id = object::new_id(ctx); // Initialize the loan struct let loan = Loan { id: loan_id, borrower, lender: 0x0, // Placeholder until matched with a lender amount: loan_amount, collateral, interest_rate, duration, start_time: 0, // Will be set when loan is approved }; // Emit loan request event (optional) transfer::emit_event(ctx, loan.clone()); loan } public fun approve_loan( lender: address, loan: Loan, ctx: &mut TxContext ) { // Check if loan is available assert!(loan.lender == 0x0, "Loan already approved"); // Match lender to the loan loan.lender = lender; loan.start_time = ctx.block_time(); // Transfer loan amount to borrower transfer::transfer_to(loan.borrower, loan.amount, ctx); } public fun repay_loan( loan: Loan, repayment_amount: u64, ctx: &mut TxContext ) { // Ensure repayment covers the principal and interest let total_due = loan.amount + (loan.amount * loan.interest_rate / 100); assert!(repayment_amount >= total_due, "Insufficient repayment"); // Transfer repayment to lender transfer::transfer_to(loan.lender, repayment_amount, ctx); // Release collateral to borrower transfer::transfer_coin(loan.borrower, loan.collateral, ctx); } public fun liquidate_loan( loan: Loan, ctx: &mut TxContext ) { // Ensure loan is overdue let current_time = ctx.block_time(); assert!(current_time > loan.start_time + loan.duration, "Loan not overdue"); // Transfer collateral to lender transfer::transfer_coin(loan.lender, loan.collateral, ctx); } }
# Project Introduction: DeFi for Wildlife Conservation 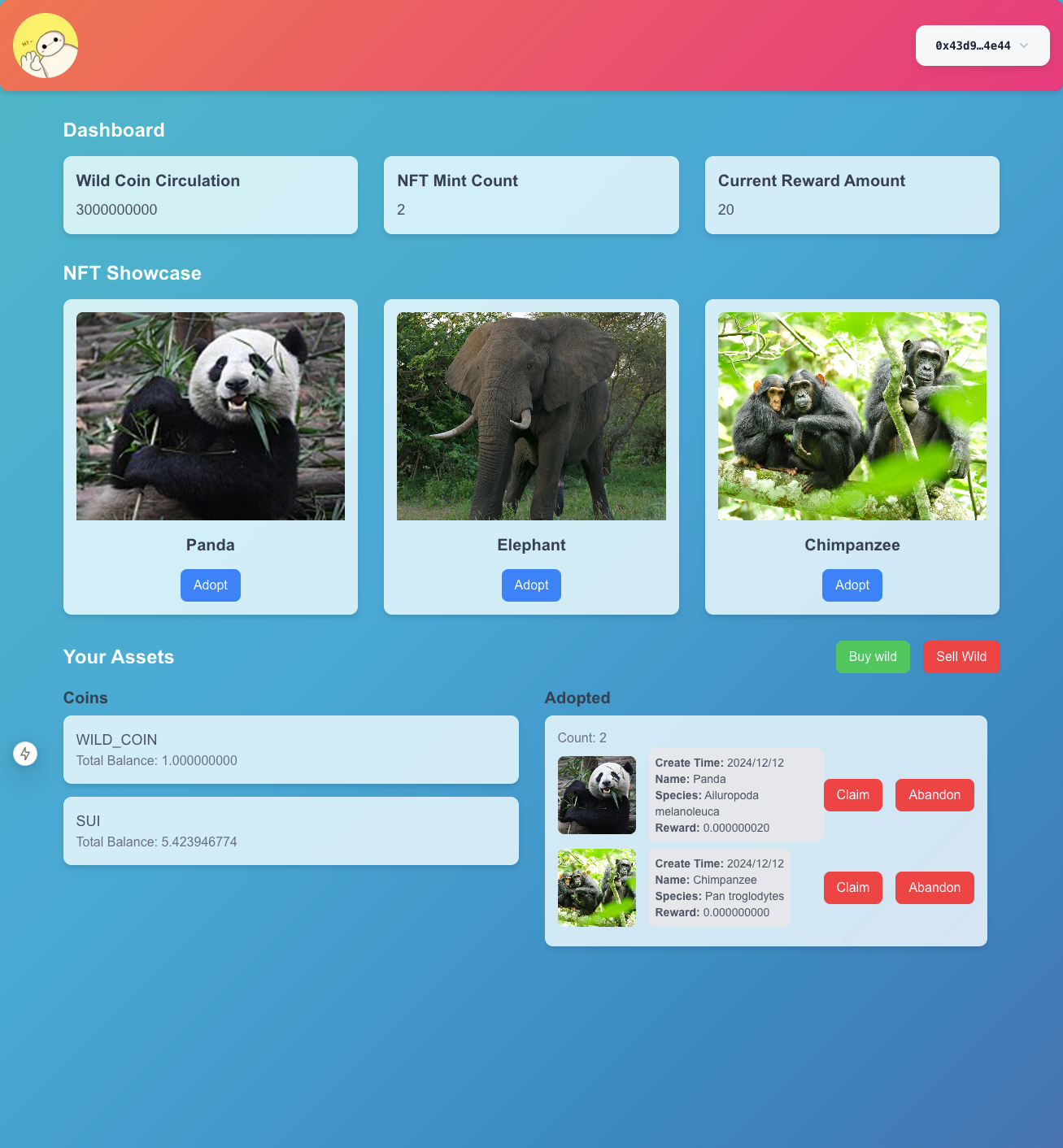 ## Project Name: WildGuard ### Blockchain Platform: Sui ### Core Token: Wild ## Vision WildGuard aims to combine decentralized finance (DeFi) with wildlife conservation, creating an innovative platform where users contribute to protecting endangered animals while engaging with DeFi-powered incentives. ## Key Features 1. **Wild Token Issuance** - **Mechanism**: Wild tokens are minted on a 1:1 basis with SUI tokens as the underlying asset. - **Stability**: SUI tokens back Wild, ensuring trust and value. - **Lifecycle**: When Wild tokens are redeemed for SUI, they are permanently burned, keeping the system balanced. - **Utility**: Wild tokens can be used to: - Adopt animal NFTs. - Vote on wildlife conservation initiatives. - Earn rewards in DeFi games. 2. **Endangered Animal NFT Adoption** - **Exclusive NFTs**: Each NFT represents an endangered species and contains detailed information about the animal. - **Adoption**: Users can adopt these NFTs by spending Wild tokens. - **Release**: Users can return the NFT to reclaim the equivalent amount of Wild tokens at any time. - **Engagement**: By owning NFTs, users contribute to conservation while enjoying an emotional connection with their chosen animals. 3. **Economic Model** **Flow of Funds**: 1. **Liquidity Mining**: - Upon NFT adoption, the equivalent SUI underlying the Wild token is deployed into liquidity mining. - This generates yield while keeping the user’s principal safe. 2. **Revenue Distribution**: - 20% for Wildlife Protection: Direct contributions to conservation organizations and initiatives. - 80% to User Rewards: Returns are allocated to a reward pool and distributed back to users. 3. **Reward Weighting**: - Calculated based on: - Animal Rarity: Rarer animals receive higher contributions. - NFT Mint Time: Older NFTs accumulate greater rewards. - Hedging Mechanism: This balances incentives, prioritizing funding for more critically endangered species. ## Advantages - **Impact-Driven**: Every NFT adoption directly supports wildlife conservation efforts. - **Zero Principal Risk**: Users gain rewards while retaining the ability to withdraw their principal at any time. - **Sustainability**: The platform sustains its mission through DeFi profits rather than relying solely on donations. - **Education**: Animal NFTs spread awareness about endangered species, fostering global conservation efforts. ## How to Get Involved - Purchase Wild tokens on the Sui blockchain. - Explore and adopt your favorite animal NFT. - Participate in voting, staking, and DeFi games while supporting wildlife conservation(in future). WildGuard unites blockchain innovation with real-world impact. Together, let’s create a sustainable future for our planet’s most vulnerable creatures!
Crab is a platform designed to help users reclaim tokens. Users can reclaim tokens and retrieve them from the platform while earning points by reclaiming tokens and marking scam tokens. These points serve as credentials for future user incentives.
ReliefChain is a blockchain-based disaster management platform built on the Sui blockchain designed to facilitate the creation, funding, and management of relief centers. It allows users to donate funds to various relief centers responsible for supporting disaster-stricken areas. Each relief center is managed by an admin who controls the funds within the center. The platform enables the transfer of funds between different centers, ensuring that resources are allocated where they are most needed. Administrators can also withdraw funds to specific recipients, ensuring efficient and transparent distribution of resources. The platform leverages blockchain technology to provide transparency, accountability, and traceability for all transactions, ensuring that donations and fund transfers are recorded on-chain for public verification.
The FreshFlow Marketplace Module is a robust smart contract designed for managing the sale and purchase of perishable goods in a blockchain-powered ecosystem. Built on the Sui Blockchain, this module ensures secure, transparent, and efficient transactions while offering dynamic pricing and seamless seller-buyer interactions. Key Features Dynamic Pricing Based on Expiration Items close to expiration (within 1 day) automatically receive a 50% discount, incentivizing sales and minimizing waste. Comprehensive Inventory Management Sellers can list items with details such as name, base price, and expiration date. Secure Purchase Mechanism Buyers can purchase items with real-time price calculation, ensuring they pay fair value based on remaining shelf life. Transparent Revenue Claim Sellers can claim accumulated earnings securely through a provider-specific capability (ProviderCap). Blockchain-Powered Events The module emits events (ItemListed and ItemPurchased) to provide transaction transparency and enable real-time tracking of marketplace activity. Key Components Structs Item: Represents a perishable good listed in the marketplace, including its unique ID, price, expiration date, and balance. ProviderCap: Grants exclusive rights to sellers for claiming earnings from a listed item. ItemListed & ItemPurchased: Events emitted during item listing and purchase respectively. Functions list_item: Allows sellers to add new items to the marketplace. purchase_item: Facilitates item purchase with real-time dynamic pricing. claim_earnings: Enables sellers to withdraw their earnings from an item’s sales. calculate_price: Computes item price dynamically based on proximity to expiration. Use Cases Grocery Stores: List and sell items nearing expiration with automated discounts. Florists: Maximize inventory turnover for flowers with a short shelf life. Electronic Retailers: Efficiently manage clearance sales for expiring warranties or limited-time offers. Conclusion The FreshFlow Marketplace Module exemplifies a modern, blockchain-powered solution for managing perishable goods. Its dynamic pricing, secure revenue management, and transparent event handling make it an essential tool for businesses aiming to reduce waste and optimize sales.
Medibridge - Service Marketplace Medibridge is a decentralized marketplace for services built on the Sui blockchain. This platform allows service providers to list their services, set prices, and receive payments in cryptocurrency. Users can pay for services and track the progress of their requests. The platform ensures secure transactions and service completion using smart contracts. Features Service Creation: Service providers can list their services with a name, price, and status. Payment Processing: Users can make payments for services with balance checks and transaction logs. Service Completion: Service providers can mark a service as completed, signaling that the service is no longer available for purchase. Proceeds Claim: Providers can claim the proceeds from completed services after payment. Technologies Sui Blockchain: For decentralized object storage and transaction management. Move Language: Smart contracts written in Move, a language for building secure and efficient blockchain programs. SUI Token: Used for payments in the marketplace. Project Structure Setup & Installation Clone the Repository Clone the Medibridge repository to your local machine: git clone https://github.com/cactusjack-28/medibridge.git cd medibridge Install Dependencies Ensure you have the Move language toolchain installed. Follow the official instructions from the Move GitHub repository to install the required tools. Deploy the Smart Contract Deploy the medibridge.move contract to the Sui blockchain. Refer to Sui documentation for specific instructions on deploying Move contracts. Run Tests Run the provided test cases to ensure that everything is functioning correctly: move test How It Works Service Creation: Service providers can create services by calling the create_service function. A service includes details like the service name, price, and balance. Making a Payment: Users can make a payment by calling make_payment, where their balance is verified, and the service's price is deducted from their balance. Completing a Service: Service providers can mark a service as completed using the complete_service function, making it unavailable for purchase. Claiming Proceeds: After completing a service, providers can claim their earnings using the claim_proceeds function. Error Handling EServiceAlreadyCompleted: The service is already completed and cannot be processed. EInsufficientBalance: The user does not have enough balance to make the payment. EInvalidServiceID: The service ID provided is invalid. EServiceNotAvailable: The service is no longer available. Contribution We welcome contributions to Medibridge! If you'd like to contribute: Fork this repository Create a new branch (git checkout -b feature-branch) Commit your changes (git commit -am 'Add feature') Push to your branch (git push origin feature-branch) Open a Pull Request License This project is licensed under the MIT License - see the LICENSE file for details. Medibridge is a decentralized service marketplace that aims to create a secure and transparent environment for users to buy and sell services using blockchain technology.
TicketPick: Real-Time Event Ticket Marketplace TicketPick is a real-time ticket marketplace built on the Dutch auction model. The platform enables event organizers to sell tickets for events such as concerts, sports matches, and more. Prices for tickets drop over time, incentivizing early buyers while providing an engaging way for users to purchase tickets at the best possible price. Features Dutch Auction Model: Prices start at a maximum value and decrease over time until a bidder accepts the price. Ticket Auctions: Event organizers (auctioneers) can create and manage ticket auctions. Real-Time Bidding: Users can place bids at the current auction price to purchase tickets. Flexible Seat Selection: Users can select specific seats based on availability. Anti-Scalping Measures: Measures to verify users and prevent scalping practices. Instant Payments: Integration with digital wallets for fast and secure payments. Requirements Move Language: The code is written using the Move programming language, deployed on the Sui blockchain. Git: For version control and managing repository updates. Sui Wallet: Integration with Sui's digital wallet for transactions. Installation Clone the repository: git clone https://github.com/soss28/ticketpick.git cd ticketpick Install Move toolchain (if you haven't already). Instructions can be found on the official Move documentation. Ensure that you have a Sui wallet setup to interact with the platform. Usage Deploying the Smart Contract Compile the Move contract and deploy it to the Sui blockchain: sui move compile --path <path-to-your-move-code> Deploy the contract: sui client publish --path <path-to-your-move-code> Interact with the contract through the Move command line or via the Sui client. Example Interaction Creating a new auction: An event organizer can create a new ticket auction by specifying the ticket price and available seats. let auction = ticketpick::auction::create(ticket, 1000) Bidding on tickets: Users can place a bid when the auction price meets their expectations: let result = ticketpick::auction::bid(auction, balance) Claiming auction proceeds: After the auction ends, the auctioneer can claim the proceeds: let claim = ticketpick::auction::claim(auction, auctioneer_cap) Error Codes EAuctionHasEnded: Auction has already ended. EAuctionHasNotEnded: Auction is still ongoing. EBalanceNotEnough: The user's balance is insufficient to place a bid. ENewPriceMustBeLower: The new price set by the auctioneer must be lower than the current price. EAuctionIDMismatch: Mismatch between the auction ID and the provided auction capability. Contributing We welcome contributions to TicketPick! If you have ideas, features, or bug fixes, please fork the repository and create a pull request. Fork the repository. Create a new branch (git checkout -b feature-name). Make your changes. Commit your changes (git commit -am 'Add feature'). Push to the branch (git push origin feature-name). Create a new pull request. License This project is licensed under the MIT License - see the LICENSE file for details. Acknowledgments The Move language and the Sui blockchain for enabling secure and scalable decentralized applications. Special thanks to the community contributors for their support.
The Automated Liquidity Rebalancer is a smart contract system that automatically adjusts liquidity positions across different pools based on market conditions. By utilizing historical data and price feeds, it optimizes yield with a dynamic fee structure that adapts to volatility. Key features include automated rebalancing driven by market volatility, a dynamic fee structure that changes with market conditions, price history tracking for volatility assessments, configurable rebalancing parameters, and event emissions for tracking significant operations.
Predicrypt is a decentralized prediction market platform on the Sui blockchain that allows users to create markets, place bets with SUI tokens, and earn rewards for accurate predictions. Key features include customizable market creation, secure authentication via zkLogin, real-time updates, community-driven resolution, automated rewards, and an easy-to-use interface.
# NexusWars: AI-Powered DeFi Strategy Game Platform A blockchain-based platform that combines algorithmic trading, game theory, and artificial intelligence to create a competitive strategy game where players build, train, and deploy AI agents to compete in simulated DeFi markets.
# SuiFund: Decentralized Scientific Research Funding Platform A blockchain-based platform that revolutionizes how scientific research is funded, validated, and monetized using the Sui Move ecosystem
created a markeplace that allow users to pay items full or pay slowly untill they complete full purchase of item and reclaim the item
created a payment system that allows users to pay for serrvices of a comapny
A decentralized event ticketing platform built on the Sui blockchain that enables secure ticket creation, sales, transfers, and validation with features like dynamic pricing, NFT benefits, and promotional codes.
# Digital Certificates and Credentialing System A blockchain-based certification and credentialing system built on the Sui network, featuring gamification, skill progression, and verifiable achievements.
This application is a decentralized bookstore management system built on the Sui blockchain. It leverages the fast, scalable, and secure features of Sui to allow users to buy, sell, and manage books in a decentralized environment. The system ensures transparency, immutability, and ownership of transactions, enabling authors and readers to interact directly without intermediaries. Features include book listings, secure transactions, and real-time updates on availability and pricing—all facilitated by the decentralized nature of the Sui blockchain.
A Sui permanent storage profile system - Supports users to customize name, description, and avatar information. - Supports users to delete their own profiles. This is very useful because it refunds some fees to users.
created an estore platform that enables users to rent and sell items on estore
## Overview This module, `stim_games::stim_games`, establishes a decentralized game store, enabling publishers to sell game licenses and users to buy, store, and view their purchased licenses. Additional features like promo codes, fee management, and a licensing verification mechanism are built in, providing a comprehensive structure for managing a blockchain-based game store. ### Components #### Struct Definitions 1. **Platform**: Represents the game platform, with attributes for owner, fee percentage, and accumulated revenue. 2. **GameStore**: A container for games and promotional codes, with features for owner management. 3. **Game**: Contains details of a game, including name, publisher, price, revenue, and a list of issued licenses. 4. **UserAccount**: Manages user-specific information such as balance and owned licenses. 5. **License**: Represents ownership of a purchased game, with details like purchase date and gifting information. 6. **Discount**: Details for promotional codes, including discount rate, expiry, and usage tracking.
Dacade is an open-sourced platform and is created in collaboration with multiple contributors. Go to the repository to start contributing.